Onboarding an Individual for Crypto Brokerage
This guide walks through setting up a Fiat and Crypto Subledger crypto brokerage integration, creating an Individual Identity and a related Account to book orders using the Order API. First, we will create an Identity to represent the user's identity on the Paxos Platform and a related Account which sets up sub-ledgering and represents the brokerage account. Then we will integrate with the Order API to set up a simple Buy, Hold and Sell integration. This guide should take around 30 minutes.
Prerequisites
- Sign up to the Paxos Dashboard in Sandbox
- Reach out to Support to provision access to the Identity API
Authenticate in Sandbox
Create an API Key on the Sandbox Paxos Dashboard with the following scopes to access the Identity, Account and Order APIs needed for this guide
identity:read_identity
identity:read_account
identity:write_identity
identity:write_account
funding:read_profile
funding:write_profile
exchange:read_order
exchange:write_order
Run below to get the access_token
from OAuth
curl --location 'https://oauth.sandbox.paxos.com/oauth2/token' \
--form grant_type=client_credentials \
--form client_id=${paxos_client_id} \
--form client_secret=${paxos_secret} \
--form scope='identity:read_identity identity:read_account identity:write_identity identity:write_account funding:read_profile funding:write_profile exchange:read_order exchange:write_order'
Confirm the response includes requisite scopes and save the access_token
to use in the request authorization header
throughout this guide.
{
"access_token": "{access_token}",
"expires_in": 3599,
"scope": "identity:read_identity identity:read_account identity:write_identity identity:write_account funding:read_profile funding:write_profile exchange:read_order exchange:write_order",
"token_type": "bearer"
}
Include the Authorization header with the bearer token on all API requests -H "Authorization: Bearer $TOKEN"
.
Creating an Individual Identity and Account
➊ Creating an Identity
To create an individual identity, we require both
- The details of the person according to the Individual Required Details Grid
- Data on who has completed identity verification (IDV) of the person.
- Passthrough
Set verifier_type
to PASSTHROUGH
and then provide details about how and when IDV was conducted for this user by setting:
passthrough_verifier_type
- the company of the IDV providerpassthrough_verified_at
- when IDV was completed for the userpassthrough_verification_id
- external id for the IDV record in the IDV provider's systempassthrough_verification_status
- the current status of IDV,APPROVED
if verifiedpassthrough_verification_fields
- the set of fields used to verify the user during IDV
All above attributes are required when verifier_type
is set to PASSTHROUGH
.
curl --location "https://api.sandbox.paxos.com/v2/identity/identities" \
--request "POST" \
--header "Authorization: Bearer ${access_token}" \
--data '{
"ref_id": "{your_end_user_ref_id}",
"person_details": {
"verifier_type": "PASSTHROUGH",
"passthrough_verifier_type": "JUMIO",
"passthrough_verified_at": "2021-06-16T09:28:14Z",
"passthrough_verification_id": "775300ef-4edb-47b9-8ec4-f45fe3cbf41f",
"passthrough_verification_status": "APPROVED",
"passthrough_verification_fields": ["FULL_LEGAL_NAME", "DATE_OF_BIRTH"],
"first_name": "Billy",
"last_name": "Duncan",
"date_of_birth": "1960-01-01",
"address": {
"address1": "123 Main St",
"city": "New York",
"province": "NY",
"country": "USA",
"zip_code": "10001"
},
"nationality": "USA",
"cip_id": "073-05-1120",
"cip_id_type": "SSN",
"cip_id_country": "USA",
"phone_number": "+1 555 678 1234",
"email": "example@somemail.org"
},
"customer_due_diligence": {
"estimated_yearly_income": "INCOME_50K_TO_100K",
"expected_transfer_value": "TRANSFER_VALUE_25K_TO_50K",
"source_of_wealth": "EMPLOYMENT_INCOME",
"employment_status": "FULL_TIME",
"employment_industry_sector": "ARTS_ENTERTAINMENT_RECREATION"
}
}'
Check the response to find the identity_id
of the newly created Identity and notice the status of the Identity is PENDING
.
Paxos will make an Onboarding Decision and asynchronously update the status to either DENIED
or APPROVED
.
{
"id": "{identity_id}",
"status": "PENDING",
"created_at": "2021-06-16T09:28:14Z",
"updated_at": "2021-06-16T09:28:14Z",
"ref_id": "{your_end_user_ref_id}",
"person_details": {
// ...
}
}
An Identity might stay in PENDING
due to being deemed high risk by Paxos. This Identity will be
required to undergo Enhanced Due Diligence. See how to automate document collection to
allow HIGH
risk customers to onboard to the crypto brokerage solution seamlessly.
➋ Wait for the Identity to be Approved
Using the above details should result in auto-approval of the Identity. You can immediately call the Get Identity endpoint
and see the summary_status
is APPROVED
.
curl --location "https://api.sandbox.paxos.com/v2/identity/identities/${identity_id}" \
--request "GET" \
--header "Authorization: Bearer ${access_token}"
- If the Identity is stuck in
PENDING
, read the Identity Status guide to help pinpoint why or reach out to Support. - Use a Webhook integration to asynchronously process
identity.approved
andidentity.denied
events to notify users when their crypto brokerage services are available (or not).
➌ Creating an Account
Now we are ready to make an Account for the Identity. An Account logically relates to a user's brokerage account, an Identity therefore can have one or many Accounts.
- Account + Profile
- Account with Beneficial Owners
Setting the create_profile
flag to true
will create a Profile for the Identity. This is how to
create accounts for a Fiat and Crypto Subledger integration.
curl --location "https://api.sandbox.paxos.com/v2/identity/accounts" \
--request "POST" \
--header "Authorization: Bearer ${access_token}" \
--data '{
"create_profile": true,
"account": {
"identity_id": "'"${identity_id}"'",
"ref_id": "{your_account_ref}",
"type": "BROKERAGE",
"description": "Brokerage account for Billy Duncan"
}
}'
For accounts with a beneficial owner we need to know about both the Identity of the brokerage account, and the Identity
of the beneficial owner. The beneficial owner should be linked during account creation by specifying the relationship in members
putting the role as a BENEFICIAL_OWNER
. The Identity for the beneficial owner should be created before
attempting the call below.
curl --location "https://api.sandbox.paxos.com/v2/identity/accounts" \
--request "POST" \
--header "Authorization: Bearer ${access_token}" \
--data '{
"create_profile": true,
"account": {
"identity_id": "'"${identity_id}"'",
"ref_id": "{your_account_ref_id}",
"type": "BROKERAGE",
"description": "Brokerage account for John Doe",
"members": [{
"identity_id": "{financial_advisor_identity_id}",
"roles": ["BENEFICIAL_OWNER"]
}]
}
}'
Booking an Order
We will use the Order API to create buy and sell orders. We recommend also taking a look at the FIX and WebSocket solutions and using the best combination of APIs for the specific use case.
➊ Fund the Account
In a complete integration, follow the Fiat Transfers Funding Flow guide to learn how to fund user assets using a Fiat Deposit.
For this guide, we will use the sandbox-only Test Funds feature in Dashboard to fund the Identity with $10,000 USD. First,
navigate to the Dashboard Landing Page and locate the Fund
button
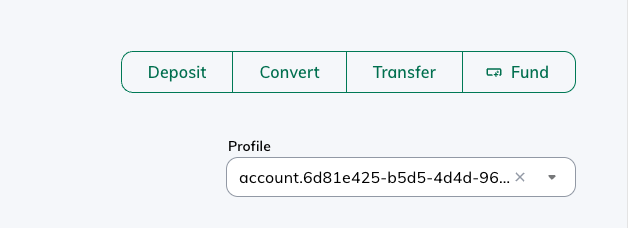
Then select the Profile related to the Account created and fund for $10,000 USD
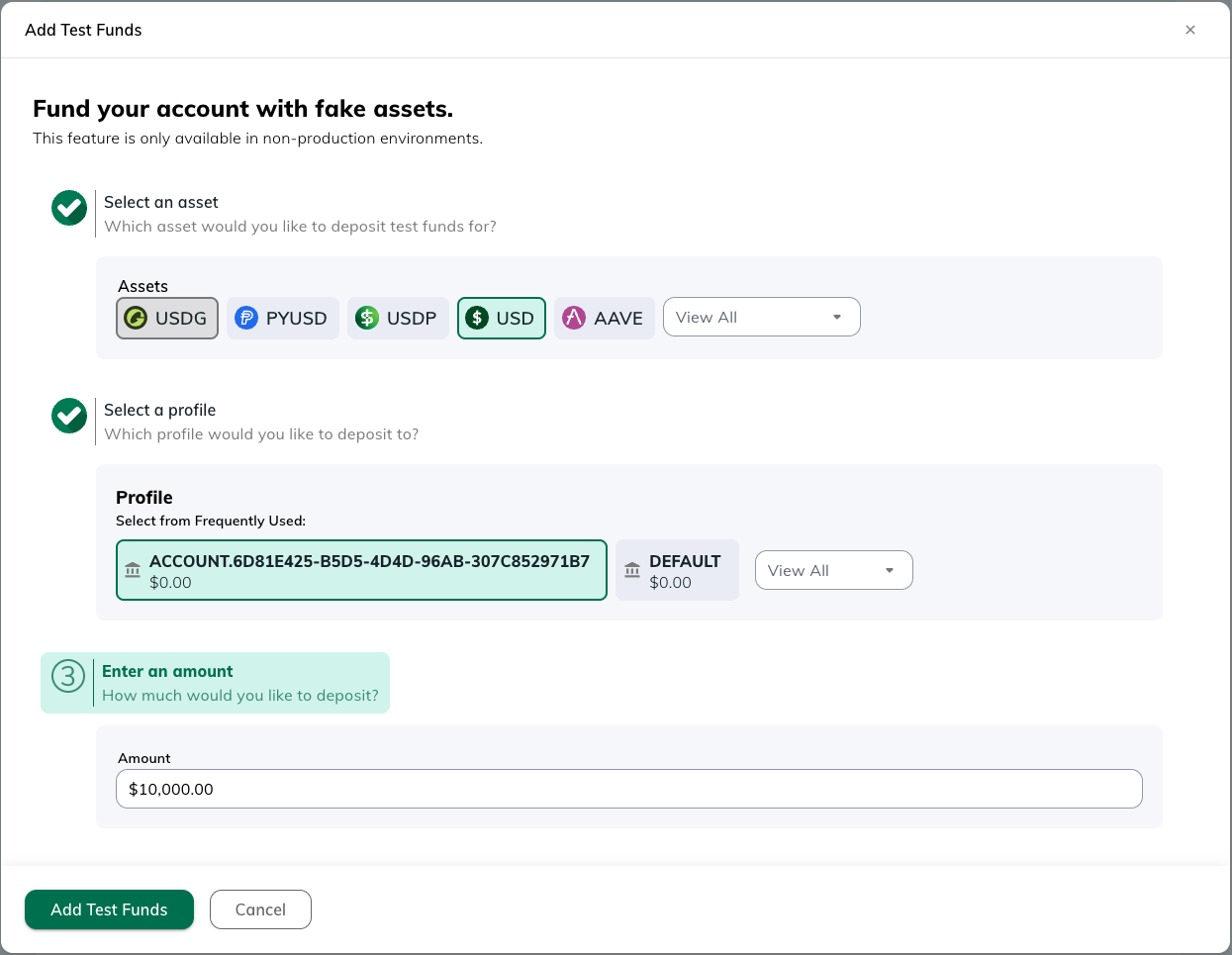
➋ Creating a Order
To book an order, specify the profile_id
which is linked to the account_id
created above (if one
exists, else the omnibus profile_id
).
- Buy
- Sell
curl --location "https://api.sandbox.paxos.com/v2/profiles/${profile_id}/orders" \
--request "POST" \
--header "Authorization: Bearer ${access_token}" \
--data '{
"side": "BUY",
"market": "ETHUSD",
"type": "LIMIT",
"price": "1814.8",
"base_amount": "1",
"identity_id": "'"${identity_id}"'",
"identity_account_id": "'"${account_id}"'"
}'
curl --location "https://api.sandbox.paxos.com/v2/profiles/${profile_id}/orders" \
--request "POST" \
--header "Authorization: Bearer ${access_token}" \
--data '{
"side": "SELL",
"market": "ETHUSD",
"type": "LIMIT",
"price": "1804.2",
"base_amount": "1",
"identity_id": "'"${identity_id}"'",
"identity_account_id": "'"${account_id}"'"
}'
You can check the status of the order by calling
curl --location "https://api.sandbox.paxos.com/v2/profiles/${profile_id}/orders/${order_id}" \
--request "GET" \
--header "Authorization: Bearer ${access_token}"
And that is it, congratulations on completing your first end-to-end crypto brokerage integration with Paxos!